Mail Order or Telephone Order Transactions
A mail order or telephone order (MOTO) transaction is a type of card-not-presented transaction in which services are ordered and paid for via telephone or mail. This payment option enables you to process transactions without the cardholder and their card being present at your place of business.
MOTO transactions are performed by keying in the card number, expiry date, CVV, and Address Verification System (AVS). The cardholder signature is not required. Merchant must make a decision about whether to approve or decline transaction based on the CVV and AVS results.
SDK version requirement:
The minimum SDK versions required are iOS 2.47 and Android 2.57.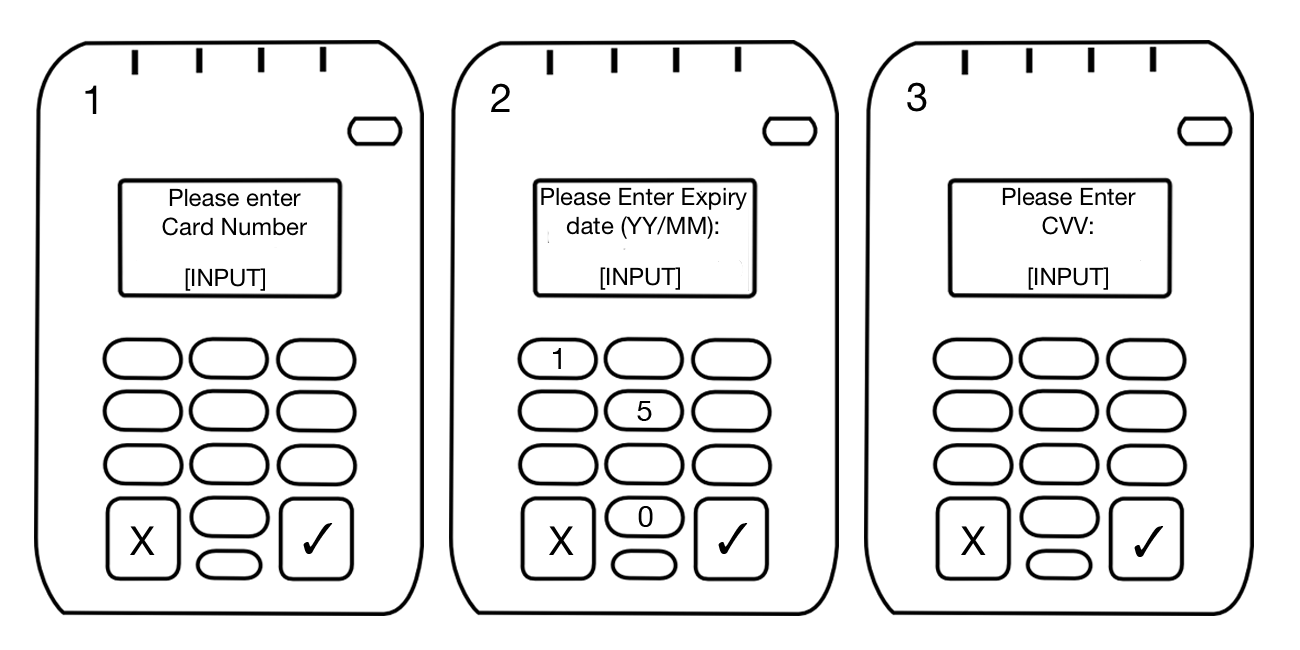
Performing MOTO Transactions
In order to process MOTO Transactions specify the transactions will be done as a MOTO workflow with
optionals.workflow = MPTransactionWorkflowTypeMoto
The transaction and the results of the transaction are just like a regular transaction.MPTransactionParametersOptionalsBlock optionalsBlock = ^(id optionals) { [optionals setSubject:@"Donuts!"]; [optionals setStatementDescriptor:@"I describe a statement"]; [optionals setCustomIdentifier:@"tx-12-don-ut"]; [optionals setMetadata:@{@"test", @"tmeta"}]; optionals.workflow = MPTransactionWorkflowTypeMoto; //MOTO transactions are like regular transactions, only input changes. }; MPTransactionParameters * params = [MPTransactionParameters chargeWithAmount:[NSDecimalNumber decimalNumberWithString:@"10.00"] currency:MPCurrencyGBP optionals:optionalsBlock]; self.transactionProcess = [self.transactionProvider startTransactionWithParameters:params accessoryParameters:[self.accessoryParametersStore currentAccessoryParameters] processParameters:[self processParameters] registered:^(MPTransactionProcess *transactionProcess, MPTransaction *transaction) { } statusChanged:^(MPTransactionProcess *transactionProcess, MPTransaction *transaction, MPTransactionProcessDetails *details) { [weakSelf notifyTransactionProcessUpdate]; } actionRequired:^(MPTransactionProcess *transactionProcess, MPTransaction *transaction, MPTransactionAction action, MPTransactionActionSupport *support) { [weakSelf notifyTransactionProcessUpdate]; [weakSelf notifyActionRequired:action support:support]; } completed:^(MPTransactionProcess *transactionProcess, MPTransaction *transaction, MPTransactionProcessDetails *details) { weakSelf.transactionProcess = nil; [weakSelf notifyTransactionProcessCompleteWithTransaction:transaction error:details.error]; }];
MOTO relies on the Address Verification System (AVS) results so it is important to understand the possible outcomes:
AVS/CVV Results | CVV correct | CVV not incorrect | CVV not checked | Transaction declined |
---|---|---|---|---|
AVS not correct | CVV Match Only | No Data Matches | No Data matches | Decline Immediately |
AVS correct | All Match | Address Match Only | Address Match Only | Decline Immediately |
Transaction declined | Decline Immediately | Decline Immediately | Decline Immediately | Decline Immediately |
The definition for the possible AVS outcomes include:
- CVV Match Only: AVS failed and CVV was performed successfully.
- Address Match Only: AVS succeeded. Card verification was performed, but was the CVV was invalid.
- All Match: Both AVS and CVV were performed successfully.
- No Data Matches: AVS and CVV were performed but both were invalid.
- Data not checked: AVS and CVV was not attempted or a security device error occurred.
Receipts
Both APPROVED and DECLINED merchant receipts must contain the following results as
statusText
:- ALL MATCH
- CVV MATCH ONLY
- ADDRESS MATCH ONLY
- NO DATA MATCHES
- DATA NOT CHECKED
If you implement ready-made receipts the
statusText
will be included. Should you require more flexibility, see the custom receipt functionality. Using custom receipts enables you to print your own receipts that include payment-related data.Testing
When you are ready to test your MOTO integration you can use the following values in zip code, Street and Amount, to emulate AVS and CVV results:
- House Number
- 999 results in not passed
- 998 results in not checked
- Zip Code
- 999 results in not passed
- 998 results in not checked
- Amount
- 999 results in cvv not passed
- 998 results in cvv not
Mail Order/Telephone Order Transactions (MOTO)
A Mail Order/Telephone Order (MOTO) transaction is a type of card-not-presented transaction in which services are paid for and delivered via telephone or mail. This payment option enables you to process transactions without the cardholder and their card being present at your place of business.
MOTO transactions are performed by keying in the card number, expiry date, CVV, and Address Verification System (AVS). The cardholder signature is not required. Merchant must be able to make a decision about whether to approve or decline transaction based on the CVV and AVS results.
SDK version requirement:
The minimum SDK versions required are iOS 2.47 and Android 2.57.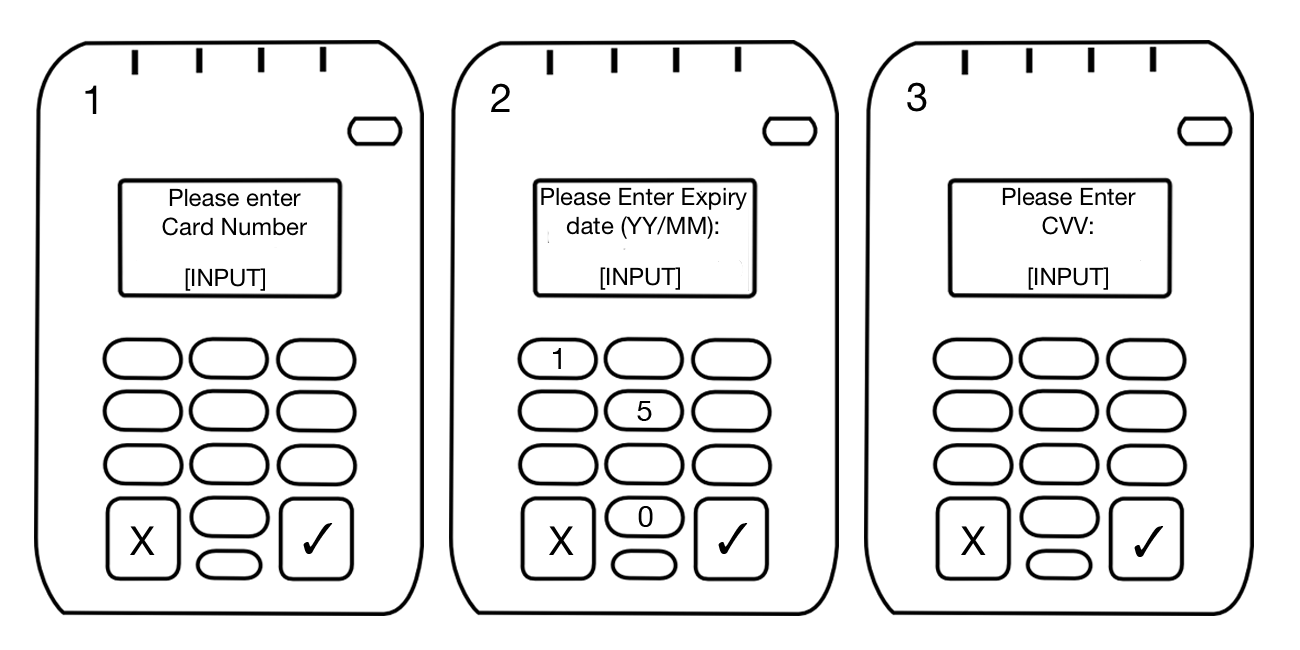
Performing MOTO Transactions
In order to process MOTO Transactions, specify the transaction workflow as MOTO with
.workflow(TransactionWorkflowType.MOTO)
The transaction and the results of the transaction are just like a regular transaction.TransactionParameters transactionParameters = new TransactionParameters.Builder() .charge(new BigDecimal(amount), currency) .workflow(TransactionWorkflowType.MOTO) .subject("My MOTO transaction") .build(); // Start the transaction as usual via TransactionProvider.startTransaction() TransactionProcess paymentProcess = transactionProvider.startTransaction(transactionParameters, accessoryParameters, new BaseTransactionProcessListener() { @Override public void onStatusChanged(TransactionProcess process , Transaction transaction, TransactionProcessDetails processDetails) { Log.d("mpos", "status changed: " + Arrays.toString(processDetails.getInformation())); } @Override public void onDccSelectionRequired(TransactionProcess transactionProcess, Transaction transaction, DccInformation dccInformation) { // not triggered for MOTO } @Override public void onCompleted(TransactionProcess process, Transaction transaction, TransactionProcessDetails processDetails) { Log.d("mpos", "completed"); if (processDetails.getState() == TransactionProcessDetailsState.APPROVED) { // print the merchant receipt Receipt merchantReceipt = transaction.getMerchantReceipt(); // print a signature line if required // and close the checkout UI } else { // Allow your merchant to try another transaction } } }); }
Since MOTO relies on the Address Verification System results, it's important to always keep in mind the possible outcomes as shown:
MOTO relies on the Address Verification System (AVS) results so it is important to understand the possible outcomes:
AVS/CVV Results | CVV correct | CVV not incorrect | CVV not checked | Transaction declined |
---|---|---|---|---|
AVS not correct | CVV Match Only | No Data Matches | No Data matches | Decline Immediately |
AVS correct | All Match | Address Match Only | Address Match Only | Decline Immediately |
Transaction declined | Decline Immediately | Decline Immediately | Decline Immediately | Decline Immediately |
The definition for the possible AVS outcomes include:
- CVV Match Only: AVS failed and CVV was performed successfully.
- Address Match Only: AVS succeeded. Card verification was performed, but was the CVV was invalid.
- All Match: Both AVS and CVV were performed successfully.
- No Data Matches: AVS and CVV were performed but both were invalid.
- Data not checked: AVS and CVV was not attempted or a security device error occurred.
Receipts
Both APPROVED and DECLINED merchant receipts must contain the following results as the
statusText
:- ALL MATCH
- CVV MATCH ONLY
- ADDRESS MATCH ONLY
- NO DATA MATCHES
- DATA NOT CHECKED
If you implement ready-made receipts, the
statusText
results will be included. Should you require more flexibility,see the custom receipt functionality. Using custom receipts enables you to print your own receipts that include payment-related data.Testing
When you are ready to test your MOTO integration you can use the following values in zip code, Street and Amount, to emulate AVS and CVV results:
- House Number
- 999 results in not passed
- 998 results in not checked
- Zip Code
- 999 results in not passed
- 998 results in not checked
- Amount
- 999 results in cvv not passed
- 998 results in cvv not checked